CinderBlocks
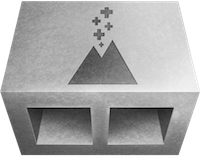
A CinderBlock is a prepackaged collection of code and libraries which implement a feature or exposes a library in Cinder. This includes bridges to libraries like OpenCV and FMOD, as well as standalone implementations of features like TUIO support. Cinder ships with several built-in CinderBlocks such as QuickTime and the LocationManager. A CinderBlock can include source, headers, libraries and any other resources it depends on.
TinderBox (Cinder's application-creation tool) is the most straightforward way to use CinderBlocks, though it is not strictly necessary and an experienced C++ user can add a CinderBlock to a project by hand. TinderBox parses the blocks directory of a Cinder installation and presents the user with a graphical interface like this:
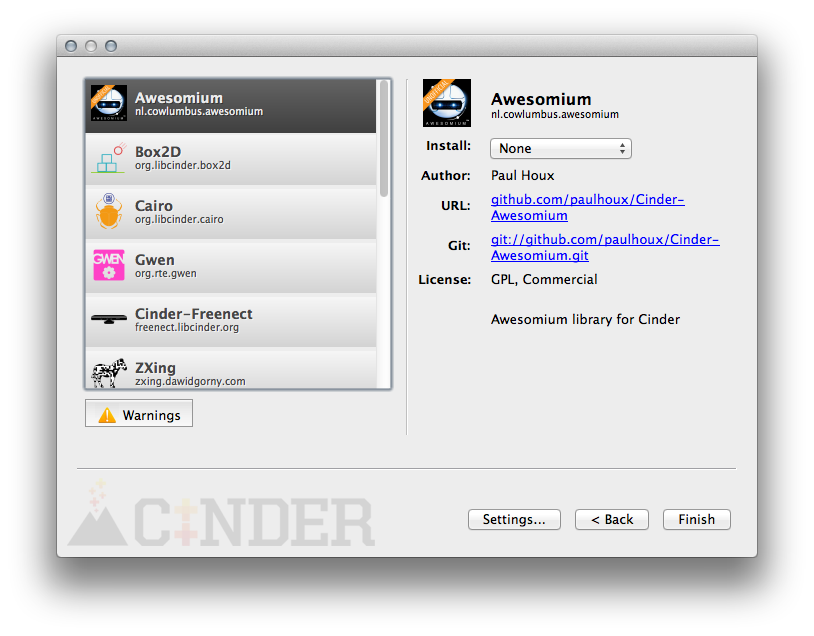
Specification
Every CinderBlock must have a file named cinderblock.xml in its root. This file enumerates the files and dependencies of the CinderBlock using XML of the following form:
<cinder>
<block>
...
<block>
<cinder>
<block>
tag supports the following attributes:
<block>
tag can contain a number of different elements. For standard files
like headers, source files, assets, etc, the structure is as follows:
<tag>
path to the file relative cinderblock.xml
</tag>
<header>include/example.h</header>
include/example.h
will be copied to <span style="font-style: italic;">project-path</span>/blocks/<em>blockName</em>/include/example.h.
<source>:
A source file, typically a .cpp or .mm file
<header>:
A header file, typically a .h file
<resource>:
A resource file. Added to Resources.rc on Windows, and
added to the Resources group and build phase in Xcode.
<asset>:
An asset file, added to the project's assets
directory. Note: Uniquely, assets are copied to the project's assets folder regardless of the install type the user has selected for the CinderBlock.<framework>:
An OS X/iOS framework (OS X/iOS-only). May also be used
with a System or SDK-relative dynamic library such as libiconv.dylib
<buildCopy>:
A file which is copied to the folder containing the build
product as part of the build process - typically a DLL or dylib.<source>
, <header>
, <resource>
,
<asset>
, <framework>
, and <buildCopy>
tags
<includePath>:
Adds a directory to the output be searched for #include
statements
#include <something.h>
rather than #include "something.h"
<frameworkPath>:
Adds a directory to the output be searched for
OS X/iOS frameworks (OS X/iOS-only)
<libraryPath>:
Adds a directory to the output be searched for
static libraries
<staticLibrary>:
Adds an OS X/iOS .a or Windows .lib static library
to the project<dynamicLibrary>:
Adds an OS X/iOS .dylib or Windows .dll dynamic library
to the project
<headerPattern>:
Adds as headers all files matching the pattern,
including wildcards (*) <headerPattern>include/*.h</headerPattern>.<sourcePattern>:
Adds as source all files matching the
pattern, including wildcards (*)
<sourcePattern>src/*.cpp</sourcePattern>.
<setting>:
Allows modification of an Xcode setting (OS X-only). The
value of the element becomes the value of the setting.
<outputExtension>:
Specifies the file extension of the build
product, i.e. ".scr" for Windows screensavers
<platform>:
Specifies an OS, compiler, build configuration or iOS SDK.
Explained further below.<supports>:
When a non-zero number of <supports> tags, specifies
which operating systems a block supports; one config per tag
<requires>:
Specifies a CinderBlock which a project template or
CinderBlock requires, identified by its reverse-dns ID
<platform os="msw">
<header>include/myLib.h</header>
<platform config="debug">
<staticLibrary>myLib_d.lib</staticLibrary>
</platform>
<platform config="release">
<staticLibrary>myLib.lib</staticLibrary>
</platform>
</platform>
Creating Template Projects
<cinder>
tag after the
<block>
<cinder>
<block>
...
<block />
<template>path/to/template.xml<\template>
...
<template>path/to/another/template.xml<\template>
</cinder>
<cinder>
<template name="OpenCV: Basic"
parent="org.libcinder.apptemplates.basicopengl">
...
</template>
</cinder>
Best Practices for Maintainers
- Include an icon: TinderBox will detect and show a cinderblock.png if present. This should be at least a 72x72 pixel PNG with an alpha channel.
- Setup a git repo: In order to allow users to include your library as a git submodule, it must have its own git repository.
- Consider minimalism: In many cases a minimal CinderBlock-specific API is preferable. An example is the Cinder OpenCV block, which serves more as a shim than as its own interface. Particularly when a library is already object-oriented, it may be the best design decision. It requires less maintenance and allows a user who is already familiar with the library to utilize her knowledge of it, rather than having to learn an "API to an API".
- Pursue loose coupling: For visually-oriented CinderBlocks, this may mean separating the render logic out into other classes or functions, particularly in the sense of not assuming an application is using OpenGL.
- Weigh binaries: In many instances a static library is unnecessary, and simply including a library's source files is adequate. This simplifies maintenance and allows the library to be used on platforms you may not have immediate access to. The CinderBlock for Box2D is an example of this technique. Other libraries, like OpenCV, must be static libraries. A natural decision maker would be whether the library requires a build system like CMake.
- Favor static over dynamic libraries: Cinder apps are designed to be self-contained whenever possible.
OS X & iOS
- Libraries should be universal binaries, supporting the i386 and x86_64 architectures on OS X and the armv7 and arm64 architectures on iOS.
- Where necessary, the C++ stdlib should be libc++, and the C++ Dialect must be C++11.
Windows
- Make sure the C Runtime is Multithreaded Debug (/Mtd) for debug and Multithreaded (/MT) for Release.
Advanced: Custom App Templates
Advanced users may want to create new App template types. An organization may have a style they'd like all applications to conform to, or you may find yourself creating the same sort of application frequently. These templates are located at Cinder/blocks/__AppTemplates. Inside you'll see a directory for each of the default application types, and you can create your own here as well.
Very advanced users may find cause to modify lower level parameters of the Xcode and Visual C++ project files TinderBox creates. If you are familiar with the internals of your compiler's project files, you can modify the files located at Cinder/blocks/__AppTemplates/__Foundation. A key attribute of these files is that the string _TBOX_CINDER_PATH_
will be replaced with the absolute file path to Cinder, and the string _TBOX_PREFIX_
will be replaced with the user-provided project name.